As a developer, you may find the process of testing your apps challenging without the right tools. The Android Studio Emulator offers a solution by allowing you to simulate various Android devices directly on your computer.
With the emulator, you can run and test your apps across multiple device configurations without needing physical hardware.
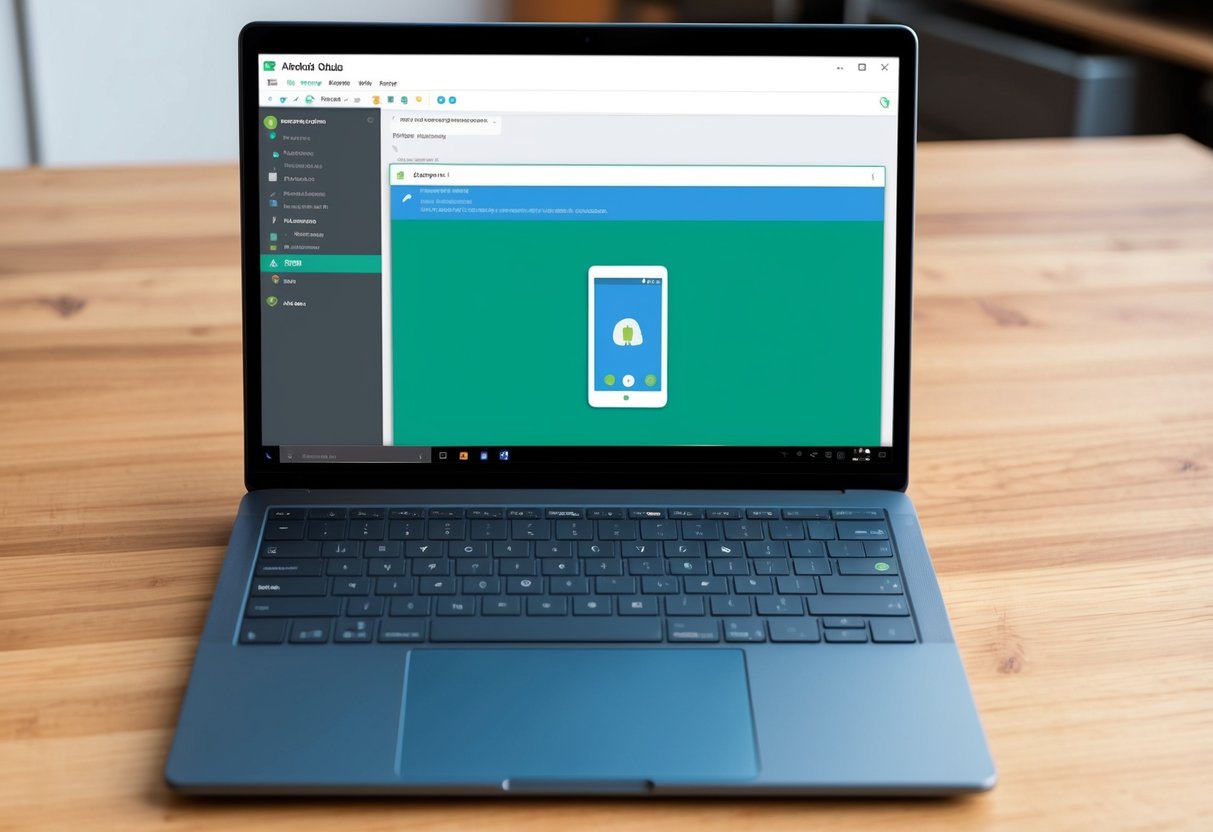
Setting up the Android Studio Emulator is straightforward, yet many users overlook its powerful features. Whether you are a beginner or an experienced developer, mastering the emulator can enhance your development efficiency. You will discover how to utilize its tools effectively to monitor your app’s performance and fix bugs swiftly.
By using the emulator, you gain the ability to test in a controlled environment, which can prevent issues when you launch your app. This aspect of Android development is vital for ensuring a smooth user experience on all devices. Exploring the full potential of the Android Studio Emulator can transform the way you approach app testing.
Setting Up the Android Studio Emulator
Setting up the Android Studio Emulator allows you to test your apps in a virtual environment. This process involves installing Android Studio, configuring the necessary tools, and managing your virtual devices.
Installing Android Studio and the Emulator
To get started, download Android Studio from the official Android Developer website. Follow the installation wizard to set it up on your computer.
During installation, make sure to check the option to install the Android Emulator. Once installed, launch Android Studio, and you will be guided through the initial setup.
After opening Android Studio, it may prompt you to install additional components. Ensure that you accept all updates to keep your installation current.
You can also verify the emulator installation by going to the “SDK Manager” within Android Studio. This manager lets you check if the emulator is ready for use.
Understanding AVD Manager and SDK Tools
The Android Virtual Device (AVD) Manager is crucial for managing your virtual devices. You can access it from the “Tools” menu in Android Studio.
In the AVD Manager, you can create, modify, and delete virtual devices.
When creating a new virtual device, you need to choose a device type, like a phone or tablet. You will also select a system image, which is an important part of the setup.
Make sure you have the correct SDK Tools installed. The SDK Tools provide essential features for building and testing your applications.
Configuring Android SDK and System Images
To configure the Android SDK, go to “File” > “Project Structure” and select “SDK Location.” Here, you will find the Android SDK Location. Make a note of this path.
Next, in the “SDK Manager,” check the “SDK Platforms” tab to select the latest Android version applicable to your project.
Then, switch to the “SDK Tools” tab to confirm that you have the latest build-tools and emulator installed.
Finally, when setting up a new AVD, choose a system image that matches the API level you want to test. This ensures compatibility and proper function of your applications.
Creating and Managing Virtual Devices
You can easily create and manage virtual devices in Android Studio using the AVD Manager. Understanding how to select system images, Android versions, and device hardware options is key to setting up your emulator successfully.
Using the AVD Manager to Create a New Device
To start, open the AVD Manager in Android Studio. You can find it by navigating to Tools > AVD Manager.
Once opened, click on Create Virtual Device. You will see a list of available device profiles.
Choose a device profile that fits your testing needs, such as a Pixel or Nexus model. After selecting a device, click Next to proceed. This step allows you to configure the virtual device settings.
Ensure that you enable hardware acceleration, as this will greatly improve performance. If you haven’t set up HAXM, the AVD Manager will guide you through that process. Once you’ve finished, click on Finish to create your new virtual device.
Selecting System Images and Android Versions
The next step involves selecting a system image for your virtual device. This is crucial because it determines which version of Android your device will run.
After clicking Next, you will see a list of available system images.
You can choose from x86 images for better performance or ARM images if you prefer. Make sure the image you select matches the Android version you want to test. You will need to download the system image if it’s not already on your system.
Make sure to select a suitable API level based on your project’s requirements. Once you have confirmed your selection, proceed to the next settings related to hardware options.
Specifying Device Hardware Options
After selecting the system image, you will configure the hardware options for your virtual device. This includes settings like RAM, screen resolution, and SD card size.
Adjust the RAM to balance performance and resource usage. A common configuration might include 1536 MB for better responsiveness.
You can also specify the screen resolution for a more accurate testing experience.
For storage, you can allocate an SD card size to simulate data storage needs. It’s often helpful to set this to at least 512 MB. Once you finalize these settings, click Finish to save your configurations, and your virtual device will be ready for use.
Running and Testing Android Applications
To effectively run and test your Android applications, you have several options. Each method allows you to engage with different features and tools within Android Studio. This section covers how to launch the emulator, run it from the command line, deploy an app, and utilize ADB for debugging and testing.
Launching Emulator from the Android Studio
To launch the Android Emulator from Android Studio, follow these steps:
- Open Android Studio: Start the application and open your project.
- Access the AVD Manager: Click on the “AVD Manager” icon in the toolbar.
- Select an AVD: Choose a pre-configured Android Virtual Device (AVD) or create a new one.
- Launch the Emulator: Click the green “Play” button to start the emulator.
The emulator may take a moment to boot up. Once running, you can interact with it just like a real device, testing your app’s features in a controlled environment.
Running the Emulator from Command Line
Running the emulator from the command line provides a flexible way to start without using the GUI. Here’s how to do it:
- Open Terminal or Command Prompt: Launch your terminal on Linux or macOS, or Command Prompt on Windows.
- Navigate to SDK Tools: Change directory to where the Android SDK is located.
- Execute the Emulator Command: Use the command
emulator -avd <your_avd_name>
Replace
<your_avd_name>
with the name of your device.
Running from the command line allows you to customize parameters and automate testing, which can improve your workflow as an app developer.
Deploying and Running an App on the Emulator
After launching the emulator, it’s time to deploy your app. To do this, follow these steps:
- Select the Emulator: Ensure your emulator is running.
- Run Your Application: Click on the “Run” button in Android Studio or use the shortcut
Shift + F10
. - Choose the Device: In the dialog that appears, select your running emulator.
Your app will compile and install automatically. Keep an eye on the emulator to see how your app performs in real-time.
Using Android Debug Bridge (ADB)
ADB is an essential tool for interacting with your emulator. It allows you to send commands and manage your app remotely. To use ADB:
Open Terminal: Launch the command line interface.
Check ADB Devices: Type
adb devices
This will show your running emulator.
Install an App: To install an APK, use
adb install <path_to_apk>
Replace
<path_to_apk>
with your app’s APK file path.Send Emulator Commands: Use ADB to run shell commands and manipulate the emulator’s state. For example, to take a screenshot, run
adb exec-out screencap -p > screenshot.png
Utilizing ADB enhances your testing capabilities, making it easier to troubleshoot issues during development.
Optimizing Emulator Performance
To get the best performance from the Android Studio Emulator, you can make specific adjustments to your settings and manage resources effectively. Attention to configuration and troubleshooting common issues will significantly improve your experience.
Configuring Emulator Settings for Performance
Start by adjusting your emulator settings for optimal performance. One important setting is hardware acceleration. Make sure to enable it to utilize your CPU’s capabilities. This can significantly speed up app loading and running times.
Setting the proper memory allocation is also crucial. Allocate enough RAM to the emulator, but leave room for your operating system. Usually, 2GB or more is recommended depending on your system’s resources.
Enable GPU emulation to leverage your graphics card for rendering. You can do this by starting the emulator with the command: ./emulator @emulatorName -gpu on
.
This setting can enhance your app’s visual performance, especially for graphic-intensive applications.
Managing Emulator Snapshots and Quick Boot
Emulator snapshots allow you to save the current state of your emulator, speeding up your workflow. Use this feature to quickly return to different project stages without needing a full cold boot.
To create a snapshot, simply pause your emulator and select the snapshot option. This can save you time in future sessions.
Consider utilizing Quick Boot, which lets your emulator start up faster by restoring from a saved state. This feature reduces load times by skipping the full boot process. Ensure that Quick Boot is enabled in your emulator settings for the best experience.
Troubleshooting Common Emulator Issues
If you encounter sluggishness or crashes, several troubleshooting steps can help.
First, check your user data and cache settings. Sometimes, excessive cached data can slow down performance.
Clear your emulator’s cache regularly.
If your emulator runs slowly, review your memory settings. Ensure it is not exceeding what your host machine can handle, as this can lead to performance drops.
Finally, keep your emulator updated. An outdated version might cause compatibility issues, which can slow down performance.
Regularly check for updates in the Android Studio SDK Manager to keep everything running smoothly.
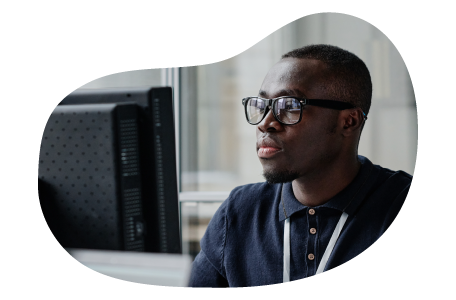
Charles Mata is an experienced app developer and educator, passionate about helping others build powerful mobile applications. He publishes in-depth guides on app development, covering Android Studio, Firebase, Google Play Console, and more. With a practical approach, he simplifies complex coding concepts, making them easy for beginners and advanced developers alike.
Charles also offers a premium website development course, where he teaches step-by-step strategies to build, optimize, and scale websites for success. Whether you’re a beginner looking to learn app development or an entrepreneur wanting to create a website, his expert insights will guide you every step of the way.