In today’s digital world, real-time chat apps are everywhere. You use them daily to stay connected with your friends, family, or coworkers. Whether it’s a quick text, sharing a photo, or getting instant updates, chat apps make communication fast and easy.
One of the most popular examples is WhatsApp. It changed the way people talk by offering instant messaging, voice and video calls, and file sharing all in one simple app. Many businesses and developers now want to create similar apps to improve customer service, build communities, or launch their own product.
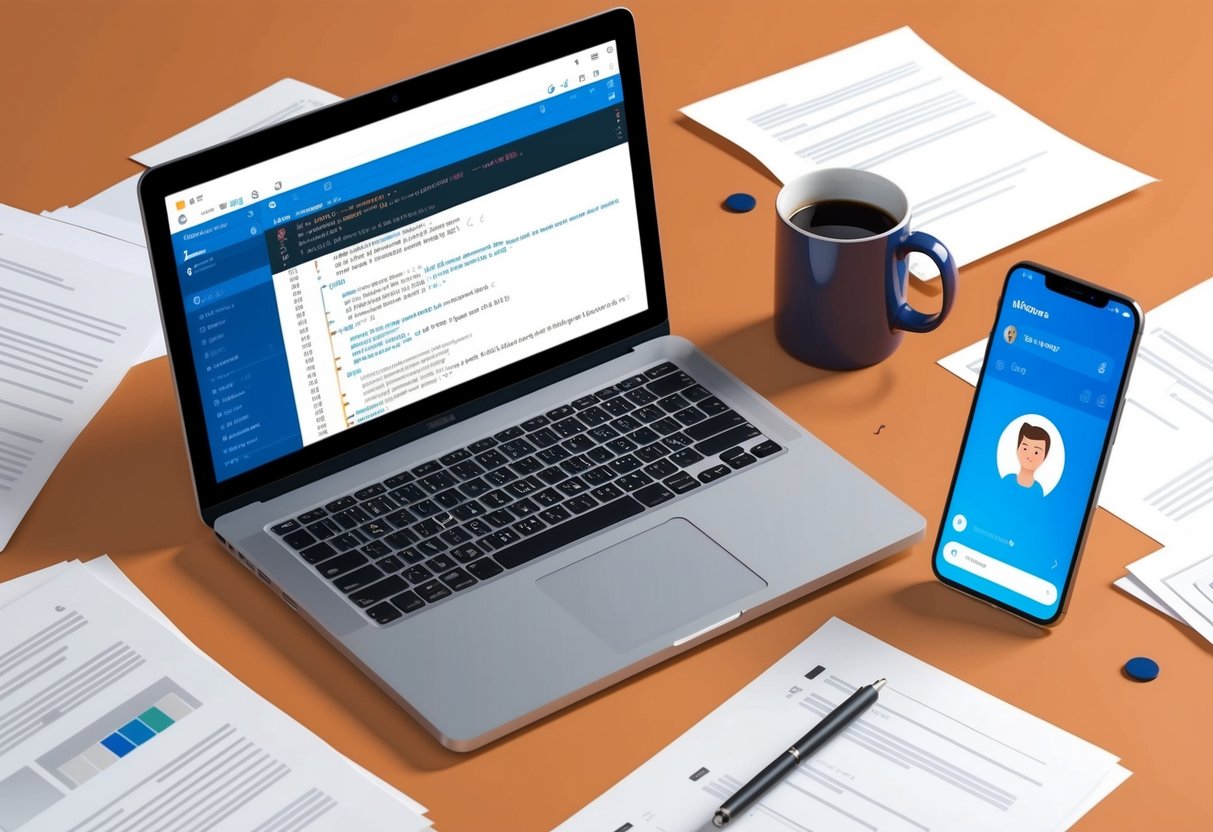
If you are wondering how to build a chat app like WhatsApp and other chat apps, this guide is for you. You will explore different ways to make one using popular tools like React, React Native, Firebase, Python, and Flutter with Dart. You’ll learn what features to include, which tech stacks to choose, and how to plan your app from start to finish.
Whether you’re building a simple messenger or trying to create something like WhatsApp, this article will help you understand the full step-by-step process.
A chat app is a software application that lets people talk to each other in real-time. It works through the internet, allowing you to send and receive messages instantly. Instead of waiting for emails or making phone calls, users can have quick and simple conversations anytime, anywhere.
What is a Chat App?
Most chat apps are designed to feel like a normal conversation. You type a message, hit send, and the other person gets it right away. Some apps even show when someone is typing or if a message has been seen. These little features make the experience feel natural and fast.
There are different types of chat apps based on where and how you want people to use them:
- Web apps: These run in your browser. You don’t need to install anything.
- Android apps: Designed for phones and tablets using the Android system.
- iOS apps: Built for Apple devices like iPhones and iPads.
- Cross-platform apps: These work on multiple systems with one codebase, often made using tools like Flutter or React Native.
When you build a chat app, you can choose what features you want to add. Some of the common ones include:
- ✓ Text messaging
- ✓ Media sharing (images, videos, audio files)
- ✓ Push notifications
- ✓ Real-time updates (instant message delivery without reloading the app)
- ✓ User authentication (login and signup)
- ✓ Group chats or private one-on-one chats
A good chat app focuses on speed, simplicity, and a smooth user experience. Whether you’re building something basic or planning to offer more advanced options later, knowing how chat apps work is the first step to creating your own.
Planning Your Chat App
Before you start building your chat app, it’s important to have a clear plan, like building a game, custom app, business app, or react app. You need to understand what kind of app you want to make and who will use it. This step helps you save time and avoid problems later.
First, decide your goal. Do you want to build a simple messaging app with just text chat? Or are you aiming for something more advanced, like a WhatsApp-like app with features such as voice calls, media sharing, and group chats? Knowing your goal helps you choose the right tools and design the app in the right way.
Next, think about the features you want to include. Every chat app has different needs, but here are some basic and optional features you can consider:
- ✓ Real-time messaging: Your app should send and receive messages instantly without refreshing the screen.
- ✓ User login and signup: Users should be able to create an account and log in securely.
- ✓ Private chats or chat rooms: You can offer one-on-one messaging or group chats depending on your app’s purpose.
- ✓ Notifications: Let users know when they receive new messages, even if they are not using the app at the moment.
- ✓ Media sharing (optional): Allow users to send images, videos, voice notes, or documents.
After choosing features, decide where you want your app to run. You have a few options:
- Web: Users can access your app through a browser on their desktop or phone.
- Mobile: Build a native app for Android or iOS.
- Cross-platform: Use a single codebase to run the app on both web and mobile. Tools like React Native and Flutter are good choices for this.
Also, during your planning, think about future updates. Maybe you want to add voice or video calls later. You don’t have to build everything in the beginning, but planning ahead gives you a strong foundation.
This is the stage where you move from just an idea to a real product. You’re not just learning how to make a chat app or how to create a chat app, but you’re shaping it based on what your users need and what technology fits best. The clearer your plan, the easier the building process will be.
How to Build a Chat App (Step-by-Step Guide Using React, Flutter, Python & Firebase)
a. How to Build a Chat App with React (Web App)
If you want to build a chat app for the web, React is one of the best choices you can make. React is a popular JavaScript library that helps you build fast and interactive user interfaces. It’s widely used by developers around the world and is well-supported with many tools, libraries, and tutorials.
When you use React to build your chat app, you have two main options for adding real-time functionality:
React with Firebase
Firebase makes it easy to build real-time apps without writing a backend. You can use Firebase Authentication for login, Firestore or Realtime Database for sending and receiving messages, and Firebase Hosting to deploy your app.
This combination works well if you are a beginner or want to build and launch your app quickly. Firebase handles the backend, so you don’t have to worry about setting up a server. You just focus on building the frontend using React.
With Firebase, you also get real-time updates automatically. When one user sends a message, the other user sees it instantly, without refreshing the page. This is perfect for chat apps where speed matters.
React with Node.js and Socket.IO
If you want more control over how your app works, you can use Node.js as your backend along with Socket.IO for real-time communication. This setup allows you to create a custom chat server and manage how data flows between users.
Socket.IO uses WebSockets, which are faster and more efficient than traditional HTTP. With WebSockets, your chat app can send and receive messages in real-time, just like apps such as WhatsApp or Messenger.
This setup is better if you already have some experience with coding and want to add custom features like typing indicators, chat rooms, or message history stored in a database like MongoDB or MySQL.
When to Use This Stack
Use React with Firebase if you want:
- A quick and simple solution
- No need to write backend code
- A modern look with a responsive interface
Use React with Node.js and Socket.IO if you want:
- Full control over chat logic
- To learn how chat systems really work
- To add more advanced and custom features
Whether you choose Firebase or a Node.js backend, using React gives your app a clean, fast, and flexible frontend. If you’re looking to learn how to build a chat app with React, this is a solid path to start with. It allows you to understand both how modern web apps are built and how real-time features work behind the scenes.
b. How to Build a Chat App with React Native and Firebase (Mobile App)
If you want to create a mobile chat app, React Native is one of the best frameworks to use. It allows you to build apps for both Android and iOS using a single codebase. This means you don’t have to write separate code for each platform, which saves you time and effort.
React Native uses JavaScript, so if you already know React for web development, you can quickly understand how React Native works. The layout and component system are quite similar, which makes it easier to switch between web and mobile development.
One of the main reasons developers choose React Native is that the apps look and feel like native apps. They run smoothly, have fast performance, and can access native features like the camera, notifications, and contacts using simple packages.
To build the backend for your chat app, Firebase is a great choice. It provides everything you need without managing your own server. With Firebase, you can handle user authentication, store and sync messages in real-time, and send push notifications. This makes your development process faster and less stressful.
Here’s how a simple setup might look using Firebase with React Native:
javascriptCopyEdit// Example of sending a message to Firebase Firestore
import { addDoc, collection } from 'firebase/firestore';
import { db } from './firebaseConfig';
const sendMessage = async (text, userId) => {
try {
await addDoc(collection(db, 'messages'), {
text: text,
sender: userId,
timestamp: new Date()
});
} catch (error) {
console.log('Error sending message:', error);
}
};
In this example, you send a message to a Firestore collection called “messages”. Firebase stores it, and other users who are listening to this collection will see the new message instantly. You don’t have to build any complex backend code for this to work.
Firebase also supports Firebase Authentication, which lets you add login and signup features using email/password, Google login, or even phone number. For push notifications, you can use Firebase Cloud Messaging (FCM) to send alerts when new messages arrive.
This stack is perfect for mobile developers who want to build and launch a real-time chat app quickly. You don’t need to worry about server-side coding, database setup, or managing hosting. Firebase takes care of it, and React Native gives you a powerful frontend for mobile.
If you’re learning how to build a chat app with React Native and Firebase, this method gives you a modern, fast, and easy way to start. You can later improve your app with features like media sharing, typing indicators, or even voice and video calls using third-party APIs.
c. How to Build a Chat App with Firebase (No Backend Code)
If you want to build a chat app without writing any backend code, Firebase is the best tool to use. It gives you ready-made services like Firestore, Firebase Authentication, and the Realtime Database, so you can focus only on the frontend.
Firestore and Realtime Database both allow you to store and sync chat messages in real-time. When one user sends a message, others can see it instantly without needing to refresh the page or reload the app.
Firebase Authentication helps you set up login and signup easily. You can allow users to sign in with email and password, Google account, phone number, or other methods. It handles user sessions and security so you don’t have to build your own login system.
This method is very useful if you are a beginner or want to launch a Minimum Viable Product (MVP) quickly. You don’t need to set up servers or manage hosting. Firebase manages everything for you in the background.
You can connect Firebase to any frontend framework such as React, Vue, Angular, or even basic HTML and JavaScript. This flexibility gives you more options depending on your skill and what kind of user interface you want to build.
If your main goal is to learn how to build a chat app with Firebase, this approach is simple, fast, and powerful. Later, if you want to grow your app or add custom features, you can move to a more complex backend.
d. How to Build a Chat App in Python (Backend App)
If you prefer to build your own backend and want full control over how your chat app works, Python is a strong choice. It is a popular programming language known for its simplicity and flexibility.
You can use Django with Channels or Flask with Socket.IO to create real-time chat features.
Django is a high-level framework that helps you build web applications fast. When you add Django Channels, it supports WebSockets, which are needed for real-time messaging.
Flask is a smaller and more flexible framework compared to Django. When used with Flask-SocketIO, it can also handle WebSockets, making it possible to build a chat app with real-time updates.
This approach is perfect if you already have some experience in backend development. It allows you to manage everything from user data to custom business logic and advanced chat features. You can also connect your Python backend to a frontend built with React, Vue, or any other framework.
If you are learning how to build a chat app in Python, you will get a deeper understanding of how messages are sent, received, and stored. This setup is not as quick as Firebase, but it gives you full freedom to design your app the way you want.
e. How to Build a Chat App UI with Flutter and Dart
If you want to build a chat app that works on both Android and iOS with a beautiful and smooth design, Flutter is a top choice. Flutter is a framework created by Google, and it uses a language called Dart.
Flutter is known for creating modern and fast user interfaces. You can use it to build the full frontend of your chat app using just one codebase. That means you write your code once, and it works on both platforms.
For the backend, you can use Firebase or Supabase. Firebase is more popular and has many ready-to-use services like Firestore, Authentication, and Cloud Messaging. Supabase is an open-source alternative that offers similar features using PostgreSQL.
With Flutter, you can easily create screens for login, chat rooms, and message input. It also supports animations and smooth transitions, which improve the overall user experience.
This setup is ideal if your main goal is to build a native-looking mobile app without writing separate code for Android and iOS. It’s also good for teams that want to move fast and focus on UI and user experience.
If you want to learn how to build a chat app UI with Flutter and Dart, you will be working with modern tools that help you deliver a high-quality mobile app in less time.
Step-by-Step Guide (Choose Any Stack)
No matter which tech stack you choose—React, React Native, Python, Flutter, or Firebase—the overall steps to build a chat app are mostly the same. You may use different tools or code, but the process stays similar. Here is a simple step-by-step guide you can follow for almost any stack.
1. Setup Project and Dependencies
Start by setting up your development environment. Choose your platform first—web or mobile. If you are using React or Flutter, create a new project using their CLI tools. For React, you can use create-react-app
, and for Flutter, use flutter create
.
Then, install the tools and libraries you need. This can include Firebase SDK, authentication libraries, or WebSocket clients like Socket.IO. Make sure your project folder is organized from the beginning to avoid issues later.
2. Create Login System
Your chat app needs a way for users to sign in. Use Firebase Authentication if you want an easy and fast solution. It supports email, Google, phone, and other login options. If you’re building a custom backend with Python or Node.js, create API routes for registration and login, and store user data securely in a database.
Once users can log in and log out, you can link their messages to their user ID or profile.
3. Build Chat UI
Now it’s time to design the chat interface. Keep it simple and easy to use. Most chat UIs have a message input field at the bottom, a send button, and a scrollable area where all messages are shown.
If you’re using React Native or Flutter, you can use ready-made UI components to build the interface. Add styling to make it clean and user-friendly. Make sure messages from different users are easy to tell apart, and show timestamps if needed.
4. Connect to Real-Time Database or Sockets
This is the part that makes your chat app come to life. Connect your app to a real-time database like Firebase Firestore or Realtime Database. These tools let you send and receive messages instantly without refreshing the screen.
If you’re using a backend with Python or Node.js, you can use WebSockets to create real-time communication. Tools like Socket.IO help you manage connections, send messages, and receive updates in real-time.
Make sure messages are saved correctly, and that both users can see them without delay.
5. Test and Deploy
Once everything is working, test your app carefully. Try sending messages, logging in and out, and checking how it works on different devices or browsers. Fix any bugs you find.
When you’re happy with the result, deploy your app. If it’s a web app, you can use Firebase Hosting, Vercel, or Netlify. For mobile apps, you can build and release them on the Google Play Store or Apple App Store.
Keep checking your app after launch to make sure it runs smoothly. You can also ask users for feedback and improve it over time.
Creating a chat app requires careful planning and setup of your development environment. This includes choosing the right technologies, installing necessary software, and configuring the server for smooth operation.
This step-by-step guide helps you understand the process of how to build a chat app from start to finish. You can use it with any tech stack, and add more features later as your app grows.
Tools and Libraries You Can Use
When building a chat app, using the right tools can save you time and make development easier. These tools and libraries help you add real-time features, handle authentication, send notifications, and even manage voice or video calls. Below are some of the most useful tools you can use depending on your tech stack and the type of app you want to build.
Firebase
Firebase is a complete backend solution from Google. It is one of the most popular tools for building chat apps, especially for beginners and small teams. With Firebase, you get:
- Firebase Authentication to handle user login and signup
- Firestore or Realtime Database to store and sync messages in real time
- Firebase Cloud Messaging to send push notifications
- Firebase Hosting to deploy your web app
You can use Firebase with React, React Native, Flutter, or even plain JavaScript. It is perfect if you want to build and launch your app quickly without managing your own server.
Socket.IO
Socket.IO is a JavaScript library that helps you build real-time applications. It uses WebSockets to keep a constant connection between users, so messages can be sent and received instantly.
If you are building your own backend with Node.js or Python, Socket.IO is a powerful tool to handle real-time messaging, typing indicators, user presence, and more.
Socket.IO is more flexible than Firebase in terms of how messages are sent and handled, but it requires more coding and setup.
Supabase
Supabase is an open-source alternative to Firebase. It gives you a backend with PostgreSQL database, real-time updates, authentication, and storage.
It is a good choice if you want more control over your data or prefer working with SQL. Supabase is still growing, but it supports many modern features needed in a chat app. You can use it with any frontend framework like React or Flutter.
Supabase is also a good option if you want to avoid vendor lock-in and use open-source tools.
Stream
Stream is a paid service that provides ready-made chat infrastructure. It offers powerful APIs and SDKs to help you build chat apps with features like:
- Group chats and private chats
- Reactions and threads
- Typing indicators and read receipts
- File sharing and media support
You don’t need to build everything from scratch. Stream takes care of backend, database, and scalability. This is useful if your project needs to launch fast or handle a large number of users.
Stream is more advanced than Firebase in terms of chat features, but it may cost more, especially if you are building a large app.
Twilio (for Calling Features)
If you want to add voice or video calling to your chat app, Twilio is a trusted platform. It provides APIs for phone calls, video calls, SMS, and verification.
You can use Twilio to build features like one-to-one voice calls, group video chats, or even customer support systems. It works with most programming languages and platforms.
Twilio is a paid service, but it offers good performance and support for real-time communication beyond just chat.
Expo (for React Native)
Expo is a framework and toolset for building React Native apps. It helps you start fast without setting up a lot of configuration. Expo supports things like:
- Camera and microphone access
- Push notifications
- File system and media uploads
- Over-the-air updates
If you are building a chat app with React Native, Expo makes your work easier by offering built-in tools and a smooth development experience. You can still eject later if you need more control or want to use custom native modules.
Common Mistakes to Avoid
When you build a chat app, it’s easy to focus only on the main features and forget some important points. To avoid problems later, here are a few common mistakes you should watch out for.
Ignoring scalability
In the beginning, your app may have only a few users. But if it grows, you will need to handle more users, more messages, and faster performance. If you don’t think about scalability early, your app might crash or become slow. Use tools like Firebase or Stream that are built to grow with your app.
Not handling user auth securely
User authentication is more than just login and signup. You need to make sure user data is safe. If you are using Firebase Auth, follow best practices. If you are building your own login system, make sure passwords are hashed and APIs are protected.
Poor UI/UX
Even if your app works well, users won’t enjoy using it if the design is confusing or hard to use. Keep your interface clean and simple. Make sure users can easily find the message box, see chat history, and get notifications. A good experience keeps users coming back.
Tips for Beginners
If you are just starting and this is your first time building a chat app, here are a few tips that can help you stay focused and avoid getting overwhelmed.
Start with Firebase or Stream API
These tools offer simple ways to add real-time messaging without writing backend code. They save you time and let you focus on learning how chat apps work.
Focus on chat feature first, not everything at once
Don’t try to add too many features at the start. Build the core messaging system first. Once that works well, you can add things like media sharing, group chats, or calling.
Test on real devices
If you are building a mobile app, test it on real phones, not just emulators. This helps you see how the app feels for real users and catch bugs that don’t show up in test environments.
Keep UI simple
A clean and easy-to-use design is better than a flashy one that confuses users. Focus on making the app functional, and you can always improve the look later.
Conclusion
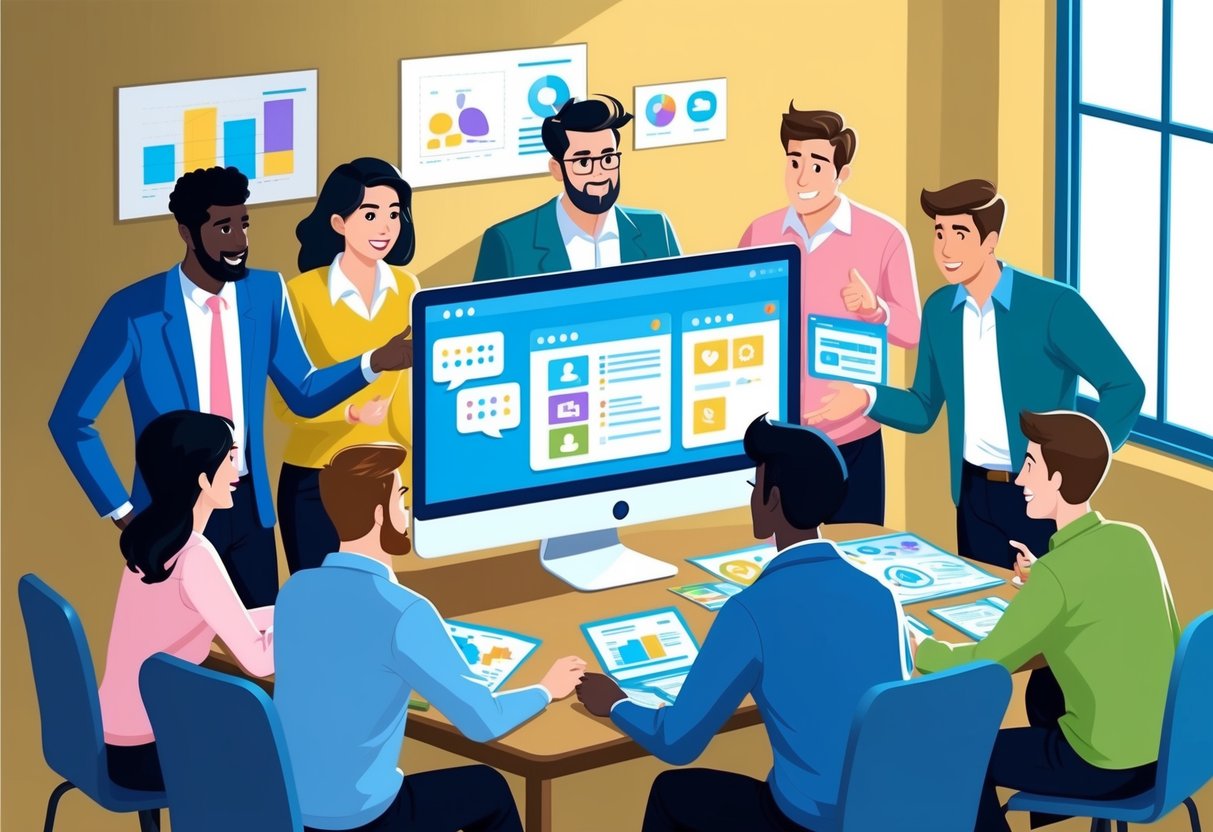
Building a chat app is a great project that teaches you how real-time systems work. You learned how to plan your app, choose the right tech stack, and use helpful tools like Firebase, Socket.IO, or Flutter. You also saw a step-by-step guide and the common mistakes to avoid.
The best tech stack depends on your skills and what kind of app you want to build. If you are just starting, Firebase or Stream can help you launch faster. If you have experience, Python or Node.js gives you more control.
Take your time, build the core features first, and improve step by step. If you want more help, feel free to check detailed tutorials on each method or leave a comment with your questions.
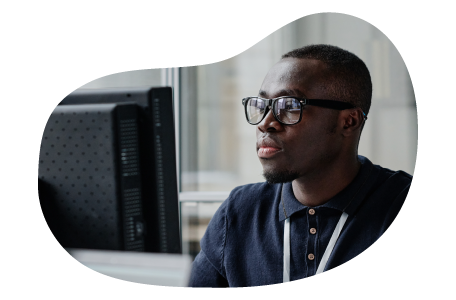
Charles Mata is an experienced app developer and educator, passionate about helping others build powerful mobile applications. He publishes in-depth guides on app development, covering Android Studio, Firebase, Google Play Console, and more. With a practical approach, he simplifies complex coding concepts, making them easy for beginners and advanced developers alike.
Charles also offers a premium website development course, where he teaches step-by-step strategies to build, optimize, and scale websites for success. Whether you’re a beginner looking to learn app development or an entrepreneur wanting to create a website, his expert insights will guide you every step of the way.